The Complete Guide to <select> and Custom HTML Dropdown Menus
Master the <select> element and custom HTML dropdown menus to create accessible, user-friendly, and dynamic form selections.
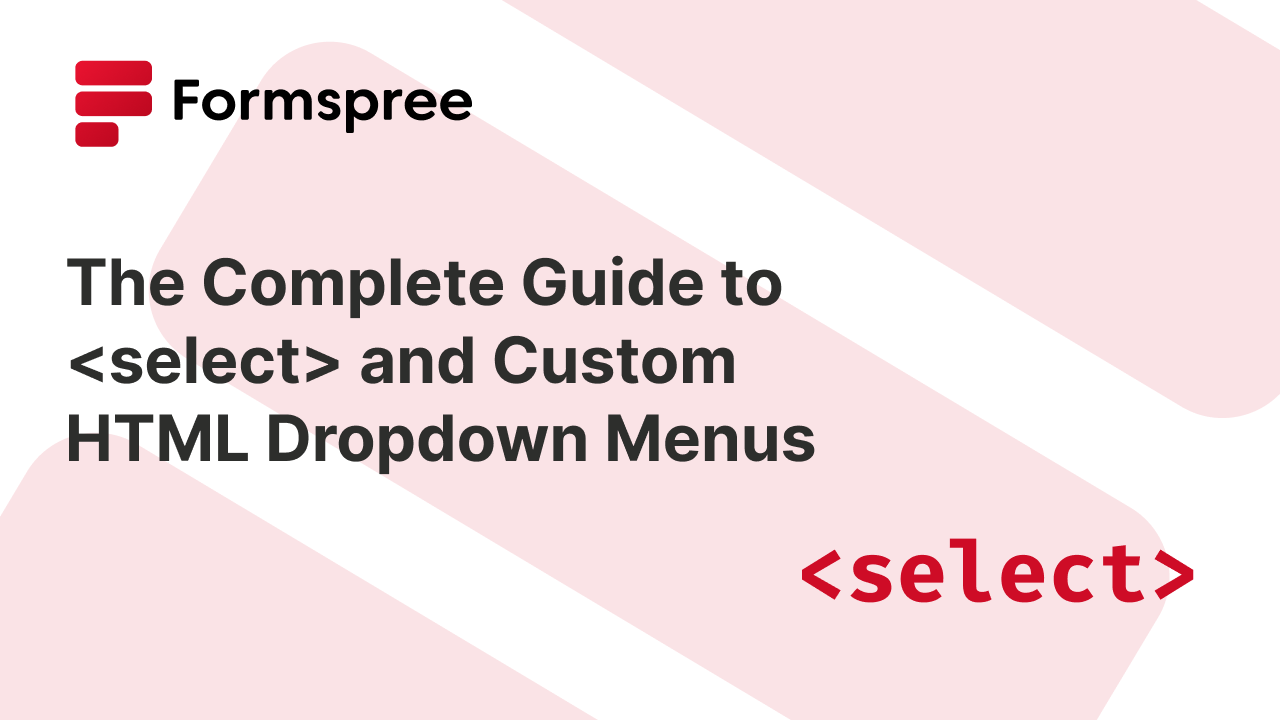
Dropdown menus are an essential component of modern web interfaces, enabling users to make selections from a predefined list of options efficiently. Whether it’s a simple form field, a complex filtering system, or a navigation element, dropdowns help streamline interactions and improve usability. However, a poorly designed dropdown can lead to frustration, hindering user experience instead of enhancing it.
In this guide, we’ll explore the different ways to create dropdown menus in HTML. We’ll start with the basics of the <select>
tag, including how to style it and add interactivity with JavaScript. Then, we’ll examine how popular frontend frameworks like React, Vue, and Vanilla JavaScript handle dropdown menus, providing best practices and library recommendations for building flexible and accessible UI components. Finally, we’ll discuss advanced design considerations, such as dependent dropdowns, searchable and filterable dropdowns, and lazy loading techniques.
By the end of this article, you’ll have a solid understanding of how to implement dropdown menus effectively, ensuring they are both functional and user-friendly. Whether you’re a beginner or an experienced developer, this guide will help you master dropdown menus for any web application.
Creating Dropdown Menus Using the <select>
Tag
The <select>
tag is the standard way to create dropdown menus in HTML. It allows users to choose from a list of options, making it ideal for forms and settings menus.
Basic Dropdown Menu with <select>
A simple dropdown menu can be created using the <select>
and <option>
elements:
<label for="fruits">Choose a fruit:</label>
<select id="fruits" name="fruit">
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="cherry">Cherry</option>
</select>
The name
attribute ensures that the selected value is sent when the form is submitted, while the id
helps with styling and scripting. The value attribute in each <option>
determines the data sent when selected.
Here’s how this code will look like:
Adding JavaScript for Interactivity
JavaScript can be used to dynamically update dropdown options or create dependent dropdowns:
<select id="countries" onchange="updateStates()">
<option value="us">United States</option>
<option value="ca">Canada</option>
</select>
<select id="states">
<option>Select a country first</option>
</select>
<script>
function updateStates() {
const country = document.getElementById("countries").value;
const statesDropdown = document.getElementById("states");
// Clear previous options
statesDropdown.innerHTML = "";
// Define state options based on the selected country
const states = country === "us"
? [
{ value: "ny", text: "New York" },
{ value: "ca", text: "California" }
]
: [
{ value: "on", text: "Ontario" },
{ value: "qc", text: "Quebec" }
];
// Populate the states dropdown
states.forEach(state => {
const option = document.createElement("option");
option.value = state.value;
option.textContent = state.text;
statesDropdown.appendChild(option);
});
}
document.addEventListener("DOMContentLoaded", updateStates);
</script>
This example updates the states dropdown based on the selected country. Here’s how it will behave:
Reviewing UX Framework Support for Dropdowns Using React, Vue, and Vanilla JavaScript
While the <select>
tag provides a simple way to create dropdowns, modern applications often require more advanced functionality, customization, and improved user experience. React, Vue, and Vanilla JavaScript offer different approaches to building dropdown menus, each with its strengths and best practices.
- React uses its component-based architecture and state management to create reusable, interactive dropdowns. Popular libraries like react-select and Downshift provide enhanced features such as searchability, accessibility, and multi-selection.
- Vue simplifies dropdown creation with its reactive data-binding and template-driven approach. Libraries like vue-select and vue-multiselect enable developers to build feature-rich dropdowns with minimal effort.
- Vanilla JavaScript is a lightweight alternative, allowing full control over dropdown behavior without dependencies. While it requires more manual work, it’s ideal for high-performance applications and projects that prioritize minimalism.
Now, let’s explore how React handles dropdown menus efficiently.
React: Best Practices and Libraries
In React applications, dropdown menus are typically implemented as controlled components, with state managing the selected value. While React’s built-in event handling makes it easy to create basic dropdowns, more advanced use cases benefit from third-party libraries that enhance functionality and accessibility.
Key Libraries for Dropdowns in React
- react-select: One of the most popular dropdown libraries, react-select provides advanced features like searchability, multi-selection, async loading, and customizable styling. It’s widely used for forms that require user-friendly selection interfaces.
- Downshift: A headless UI library that offers full control over dropdown rendering while ensuring accessibility. Unlike react-select, Downshift doesn’t impose specific styles, making it ideal for highly customized dropdown menus.
- @headlessui/react: A collection of unstyled, accessible UI components, including dropdowns, designed to work seamlessly with Tailwind CSS or custom styles. This is useful for teams that want complete design flexibility while maintaining good UX practices.
- React-aria: Developed by Adobe, this library provides accessible dropdown components with keyboard and screen reader support, making it a great choice for applications with strict accessibility requirements.
- Radix UI: A set of accessible, unstyled components that work well for dropdown menus needing enhanced control over animations, state management, and keyboard navigation.
Best Practices for React Dropdowns
- Prioritize accessibility: Use ARIA attributes and keyboard navigation to improve usability. Libraries like Downshift and React-aria help ensure dropdowns are screen reader-friendly.
- Optimize for performance: Large dropdowns should implement lazy loading or virtualized lists to prevent slow rendering. react-window and react-virtualized can help optimize dropdowns with thousands of items.
- Use controlled components as much as possible: Manage dropdown selections via state (useState or Redux) to keep the UI predictable and maintainable.
- Customize UI and styling: Avoid relying on default browser styles for
<select>
elements. Instead, use libraries like Radix UI or @headlessui/react to create dropdowns that match the application’s design system.
Vue: Best Practices and Libraries
Vue’s reactive data-binding and component-based architecture make it a great choice for building flexible dropdown menus. Whether you need a simple <select>
menu or a fully interactive custom dropdown, Vue’s ecosystem offers powerful tools to enhance functionality and user experience.
Key Libraries for Dropdowns in Vue
- vue-select: One of the most widely used dropdown libraries for Vue, vue-select provides features like searchability, multi-selection, and tagging. It offers a user-friendly experience while maintaining accessibility.
- vue-multiselect: A highly customizable dropdown component that supports multi-selection, asynchronous option loading, and filtering. It is ideal for applications requiring advanced selection features.
- @headlessui/vue: A set of unstyled components that provide full control over dropdown behavior while maintaining accessibility. This is perfect for developers who want to build fully customized dropdowns without sacrificing UX best practices.
- Vant: A UI framework optimized for mobile applications, offering dropdown components with smooth animations and mobile-friendly interactions. It’s a great choice for Vue projects targeting mobile users.
- Element Plus and PrimeVue: These UI component libraries provide feature-rich dropdown components as part of a larger set of prebuilt UI elements, making them great for teams looking for a cohesive design system.
Best Practices for Vue Dropdowns
- Use Vue’s reactivity: Bind dropdown values using
v-model
to ensure seamless two-way data binding. This keeps the UI and state in sync automatically. - Enhance user experience with search and filtering: When dealing with long lists, use libraries like vue-select or vue-multiselect to enable search and filtering, improving usability.
- Optimize performance for large datasets: Use lazy loading techniques to prevent performance issues when dealing with thousands of dropdown options. Libraries like vue-virtual-scroller can help efficiently render large lists.
- Ensure accessibility: Vue’s custom dropdown implementations should include keyboard navigation and ARIA attributes to accommodate all users. Libraries like @headlessui/vue provide accessible dropdown components out of the box.
- Customize styling for a cohesive UI: Avoid default browser styling for
<select>
elements and instead use CSS frameworks like Tailwind CSS or SCSS to design consistent dropdowns. Frameworks like Element Plus and PrimeVue also provide themeable dropdown components. - Use Vue’s slots for flexible dropdown content: Custom dropdowns often require more than just a list of text options. Utilizing Vue’s slot system allows you to insert images, icons, or other UI elements inside the dropdown menu.
Vanilla JavaScript: Simplicity and Flexibility
For projects that require minimal dependencies, high performance, or full control over dropdown behavior, Vanilla JavaScript provides a powerful way to create custom dropdown menus. While frameworks like React and Vue simplify state management and UI updates, using plain JavaScript allows developers to build lightweight, highly optimized dropdowns without the overhead of additional libraries.
Key Considerations for Dropdowns in Vanilla JavaScript
- Event-driven interactivity: Unlike frameworks that offer built-in state management, dropdowns in Vanilla JavaScript rely on event listeners (click, change, and keydown) to handle user interactions.
- Minimal dependencies: Custom dropdown implementations in Vanilla JavaScript can help avoid the bloat of third-party libraries, making them ideal for performance-critical applications.
- Complete design and functionality control: Without a framework imposing restrictions, developers can fully customize dropdown appearance, animations, and behavior using CSS and JavaScript.
- Scalability challenges: While custom dropdowns offer flexibility, managing large datasets, keyboard navigation, and accessibility features requires additional work compared to using prebuilt components in frameworks.
Best Practices for Dropdowns in Vanilla JavaScript
- Use semantic HTML whenever possible: If a simple dropdown suffices, stick to the
<select>
element instead of reinventing the wheel with<div>
elements. - Enhance UX with animations and transitions: CSS animations (
opacity
,transform
, orheight
) can improve dropdown visibility and responsiveness. JavaScript’srequestAnimationFrame
can further optimize performance. - Implement keyboard and accessibility support: Custom dropdowns should support Tab, ArrowUp, ArrowDown, and Enter key navigation, along with ARIA attributes to improve accessibility.
- Optimize for large datasets: Lazy loading or virtualization techniques help prevent performance issues when dealing with thousands of dropdown options. For example, dynamically inserting options into the DOM only when needed reduces rendering overhead.
- Debounce input events in searchable dropdowns: If implementing a searchable dropdown, use a debounce function to prevent excessive event firing while users type.
- Handle click-outside events properly: Custom dropdowns should close when the user clicks outside the menu. Adding an event listener to document and checking event targets can prevent dropdowns from staying open unnecessarily.
Alternatives to Custom Vanilla JavaScript Dropdowns
For projects requiring advanced features without a framework, libraries like Tippy.js (for dropdown tooltips), Choices.js (for searchable and multi-select dropdowns), and SlimSelect (lightweight replacement for <select>
) provide ready-made solutions with enhanced functionality.
Vanilla JavaScript remains a great option when you need a lightweight, fully customizable dropdown without dependencies. However, for large-scale applications, frameworks like React and Vue can simplify state management, accessibility, and advanced interactions.
Advanced Use Cases of <select>
While <select>
is simple to implement, it can be adapted for advanced use cases by combining it with JavaScript and CSS.
- Dependent Dropdowns: Dependent dropdowns allow users to make hierarchical selections, such as choosing a country and dynamically displaying states or provinces. This is commonly used in e-commerce and registration forms.
- Searchable Dropdowns: Enhancing
<select>
elements with a search feature allows users to filter options dynamically. Libraries like Select2 or react-select make it easy to implement searchable dropdowns with better styling and accessibility. - Filterable Dropdowns: A filterable dropdown categorizes options, allowing users to refine selections based on specific criteria (e.g., product categories or tags).
- Lazy Loading in Dropdown Menus: When dealing with large datasets, lazy loading optimizes performance by loading options only when needed. This is useful for locations, large product catalogs, or extensive lists in enterprise applications.
Conclusion
Dropdown menus are a fundamental part of web interfaces, helping users navigate options efficiently. In this guide, we explored multiple approaches to building dropdown menus. We covered how to use the <select>
tag effectively, including styling and interactivity enhancements with JavaScript. We also reviewed how different frontend frameworks—React, Vue, and Vanilla JavaScript—support dropdowns, highlighting best practices and popular libraries that simplify implementation. React’s component-driven approach, Vue’s reactive system, and Vanilla JavaScript’s flexibility each offer unique advantages depending on project needs.
For simple forms, <select>
is often sufficient, but for complex use cases—like searchable, multi-select, or dynamically updated dropdowns—frameworks and libraries such as react-select, vue-multiselect, or Choices.js provide powerful enhancements.
Choosing the right method depends on your project’s complexity, performance requirements, and design goals. By following best practices and using the right tools, you can create dropdowns that are not only functional but also seamless and user-friendly.